String Interpolation in Angular
In the previous chapter, we covered data binding and learned about one-way data binding. We achieve this through:
- String Interpolation
- Property Binding
Basic interpolation we have seen in previous chapter. Here we will create an object and will show there properties on the web page. We can use arithmetic operations also within the {{}}
Updating the Component
Inside app.component.ts
, we define properties:
export class AppComponent {
title = 'shopping-kart'
product={
name: 'Samsung S20',
price: 1000,
color:'Blue',
discount: 5
}
}
Using String Interpolation
In app.component.html
, we use string interpolation:
<h1>Name: {{ title }}</h1>
<p>Name: {{ product.name }}</p>
<p>Price: ${{ product.price }}</p>
<p>Color: {{ product.color }}</p>
<p>Discounted Price: ${{ price - (product.price * product.discount / 100) }}</p>
Calling Methods Inside String Interpolation
We can create a method to calculate the discounted price:
getDiscountedPrice(): number {
return this.product.price - (this.product.price * this.product.discount / 100);
}
Update the view template:
<p>Discounted Price: ${{ getDiscountedPrice().toFixed(2) }}</p>
Using Ternary Operators
We can check stock availability:
<p>{{ product.inStock > 0 ? 'Only ' + product.inStock + ' items left' : 'Out of Stock' }}</p>
Here is the complete code where we have used interpolation
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'shopping-kart'
product={
name: 'Samsung S20',
price: 1000,
color:'Blue',
discount: 5,
inStock:10
}
getDiscountedPrice(): number {
return this.product.price - (this.product.price * this.product.discount / 100);
}
}
app.component.html
<h1>{{title}}</h1>
<p>Name: {{ product.name }}</p>
<p>Price: ${{ product.price }}</p>
<p>Color: {{ product.color }}</p>
<p>Discounted Price: ${{ product.price - (product.price * product.discount / 100) }}</p>
<p>Discounted price from method: ${{ getDiscountedPrice().toFixed(2) }}</p>
<p>{{ product.inStock > 0 ? 'Only ' + product.inStock + ' items left' : 'Out of Stock' }}</p>
Output
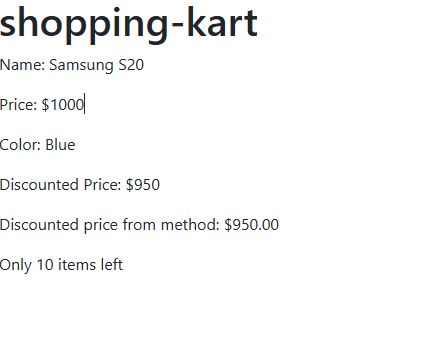
Conclusion
String interpolation allows dynamic content rendering in Angular by embedding expressions inside double curly braces {{ }}
. It helps in displaying variables, evaluating expressions, and calling methods directly in the template.
If you have any questions, feel free to ask. Thank you for reading!
If you have any query or question, please contact through below form. Our team will be in touch with you soon.
Please provide your valueable feedback or suggession as well.