Angular Component
A component is a key feature of an Angular application. Angular is a component-based framework used to build client-side applications.
A component is essentially a small piece of the user interface (UI). An Angular application is made up of multiple components that work independently and are combined to create a full application.
Structure of Components
Every Angular application has at least one root component, typically called the AppComponent
. This root component contains child components, forming a tree-like structure.
For example, a simple web application can be divided into multiple components:
- Header Component
- Navbar Component
- Sidebar Components
- Main Content Component
- Footer Component
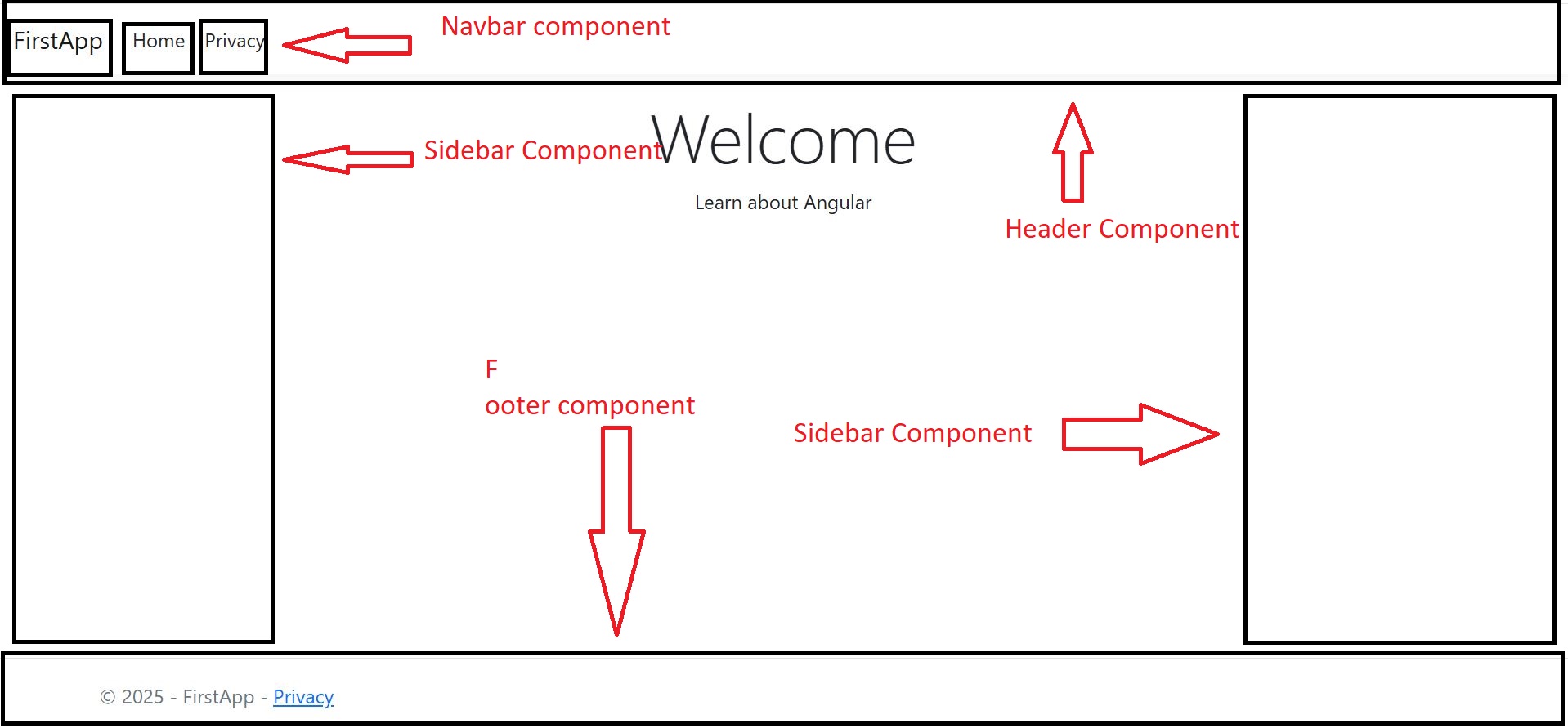
Each of these components can have their own child components, creating a structured and modular UI.
Creating a Component
to make things modular. We will create a folder and then create a componnet in that.
create a component in Angular, follow these steps:
- Create a TypeScript class and export it.
- Decorate the class with
@Component
decorator. - Declare the component in the main module file
app.module.ts
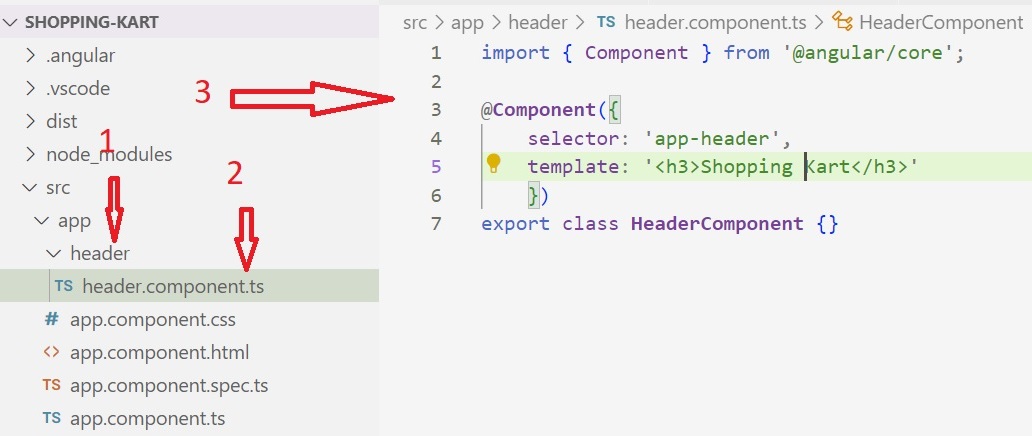
import { Component } from '@angular/core';
@Component({
selector: 'app-header',
template: '"<h3>eCart</h3>",
"price": 100'
})
export class HeaderComponent {}
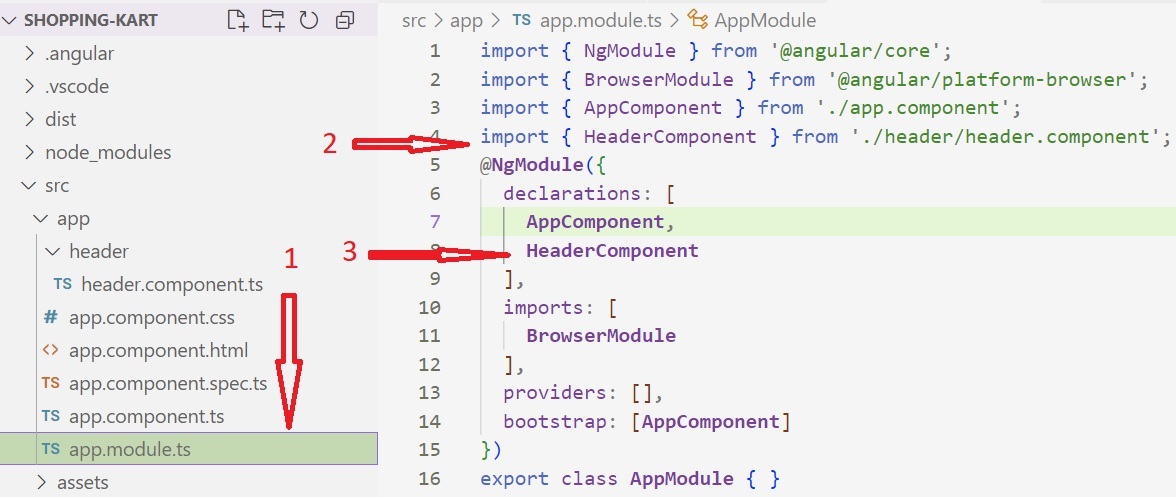
import { HeaderComponent } from './header/header.component';
@NgModule({
declarations: [HeaderComponent],
bootstrap: [AppComponent]
})
export class AppModule {}
Using a Component
To use a component, include its selector inside another component’s template. Here we will be using in app.componnet.html
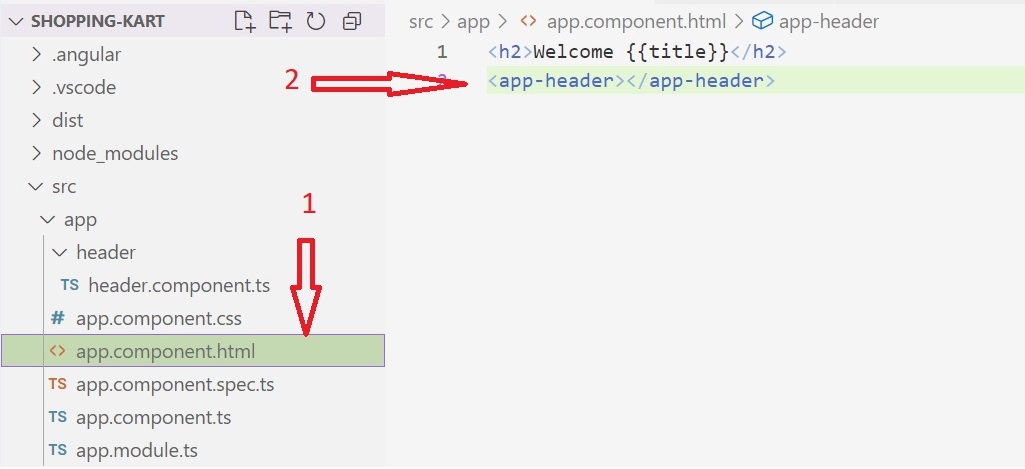
<app-header></app-header>
Component Templates
Components define their HTML using a view template. There are two ways to define a template:
- Using the
template
property (for short HTML content). - Using the
templateUrl
property (for larger HTML content stored in a separate file).
Template
template
property is generally used when we want to add some small peice of html on the page. This is done in inline way. In a single line we can define the html code. The example above we have seen, is using the inline template.
Template Url
templateUrl
In this case we are creating a separate html file and add all the html there and then use in templateUrl property. That html will be displayed where the component selector is used
We will be creating html file in same header folder with name header.component.html. We will nvabar in that.
@Component({
selector: 'app-header',
templateUrl: './header.component.html'
})
export class HeaderComponent {}
header.component.html
<div class="shoppingkart--header">
<div class="shoppingkart-logo">
shoppingkart
</div>
<div class="shoppingkart--header--nav">
<div class="shoppingkart--top--bar">
<a href="#">Help</a>
<a href="#">Exchange & Returns</a>
<a href="#">Order Tracker</a>
<a href="#">SignUp / Login</a>
</div>
<div class="shoppingkart--bottom--bar">
<div class="shoppingkart-menu">
<a href="#">Home</a>
<a href="#">Products</a>
<a href="#">About</a>
<a href="#">Contact</a>
</div>
<div class="shoppingkart--search-bar">
<input type="text" class="shoppingkart-search-box" placeholder="Search">
<button class="shoppingkart--search--btn">
<i class="fa fa fa-search" aria-hidden="true"></i>
</button>
</div>
</div>
</div>
</div>
Dont worry about the classes and fa fa icons applied on html elements in above code. We will learn styling in next chapter.
Conclusion
Components are the building blocks of Angular applications. They provide modularity, reusability, and a structured way to develop complex UIs. Using @Component
decorator, we can define and manage components efficiently.
If you have any questions, feel free to ask. Thank you for reading!
If you have any query or question, please contact through below form. Our team will be in touch with you soon.
Please provide your valueable feedback or suggession as well.